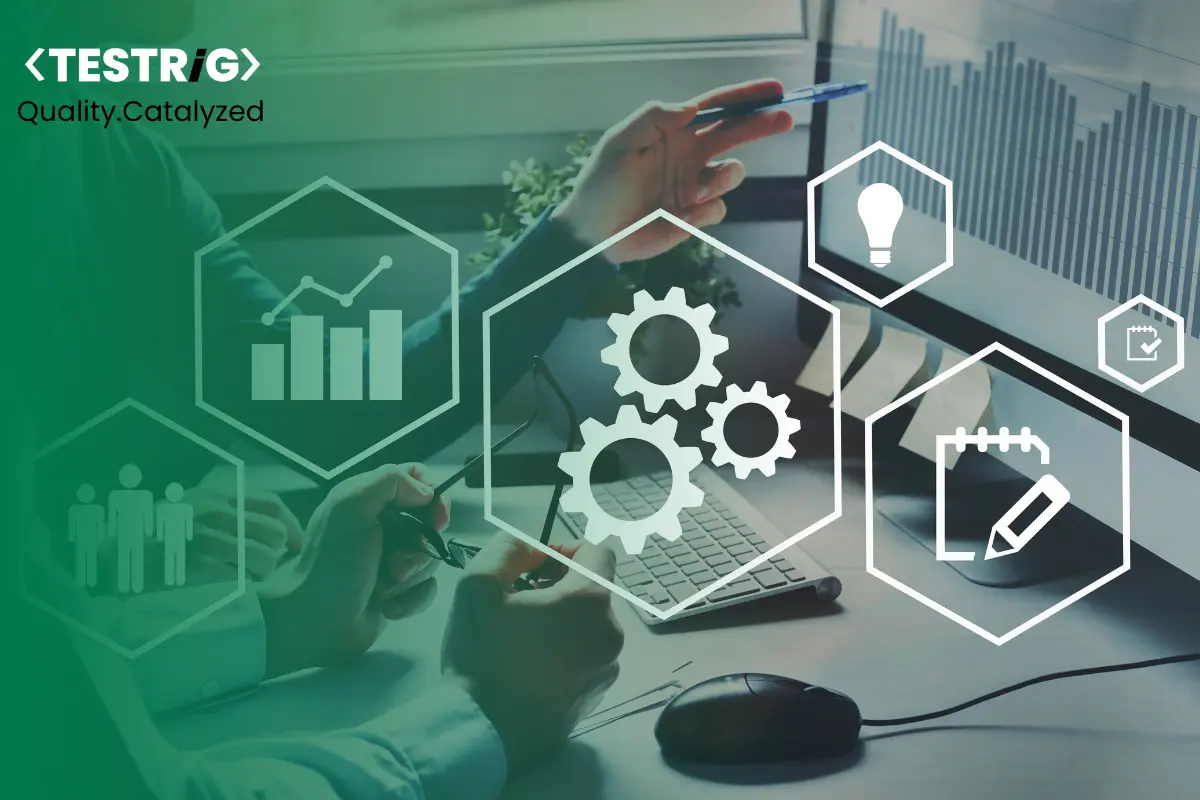
As digital experiences grow increasingly sophisticated, ensuring a web application’s user interface performs flawlessly across browsers and devices has become mission-critical. Automated UI testing has stepped up as a cornerstone of modern quality assurance—enabling faster feedback loops, reduced manual effort, and higher test coverage in agile and DevOps environments.
At the forefront of this evolution is Selenium 4—a powerful, open-source automation framework that continues to set the standard for cross-browser UI testing. More than just an upgrade, Selenium 4 brings a new era of streamlined automation with features like:
- W3C WebDriver Compliance for improved browser compatibility and stability
- Built-in Driver Management, removing the need for external driver setup
- Improved Relative Locators, allowing more intuitive element targeting
- Native Support for Chrome DevTools Protocol (CDP) for advanced test capabilities like network mocking and performance analysis
Selenium’s modular design, vibrant ecosystem, and broad community adoption make it a preferred tool not just for testers, but for developers integrating automation into the CI/CD pipeline. Whether you’re building robust test suites for enterprise platforms or validating rapid UI changes in a startup environment, Selenium 4 equips teams to deliver high-quality user experiences with confidence.
This blog takes a deep dive into automated UI testing with Selenium—from how it works under the hood, to advanced setup strategies, and best practices that address common challenges in modern development environments.
What is Automated UI Testing in Selenium?
Automated UI testing with Selenium refers to simulating end-user interactions (clicks, inputs, navigation) on web interfaces to verify application behavior. It shifts the testing from manual testers to code-based execution—ensuring faster feedback cycles and higher test coverage.
Unlike API testing, UI testing ensures:
- Layouts render correctly across resolutions and devices
- Functional flows behave as expected (e.g., login, checkout)
- JavaScript-heavy features (AJAX, dynamic content) load reliably
Selenium isn’t a test framework—it’s a browser automation toolkit. Real test automation requires orchestration through test runners, design patterns, and integrations with test lifecycle tools.
How Selenium UI Testing Works Behind the Scenes
At its core, Selenium WebDriver acts as a communication layer between your test scripts and the browser, using the W3C WebDriver protocol to execute commands like clicks, inputs, and validations.
With Selenium 4, WebDriver fully adheres to this standard and introduces Selenium Manager—a built-in tool that automatically handles browser driver setup, eliminating manual configuration in most cases.
Key Components:
- Test Script (e.g., Java/Python) – Contains user simulation logic.
- WebDriver API – Converts test logic into HTTP commands.
- Browser Driver (e.g., ChromeDriver) – Receives commands and executes actions.
- Browser – Renders the application and reacts to commands (clicks, typing).
This architecture enables:
- Cross-browser execution
- Real-device and headless testing
- Support for parallel/distributed testing using Selenium Grid or cloud providers
Example:
Test Code (Java) → WebDriver → ChromeDriver → Chrome Browser
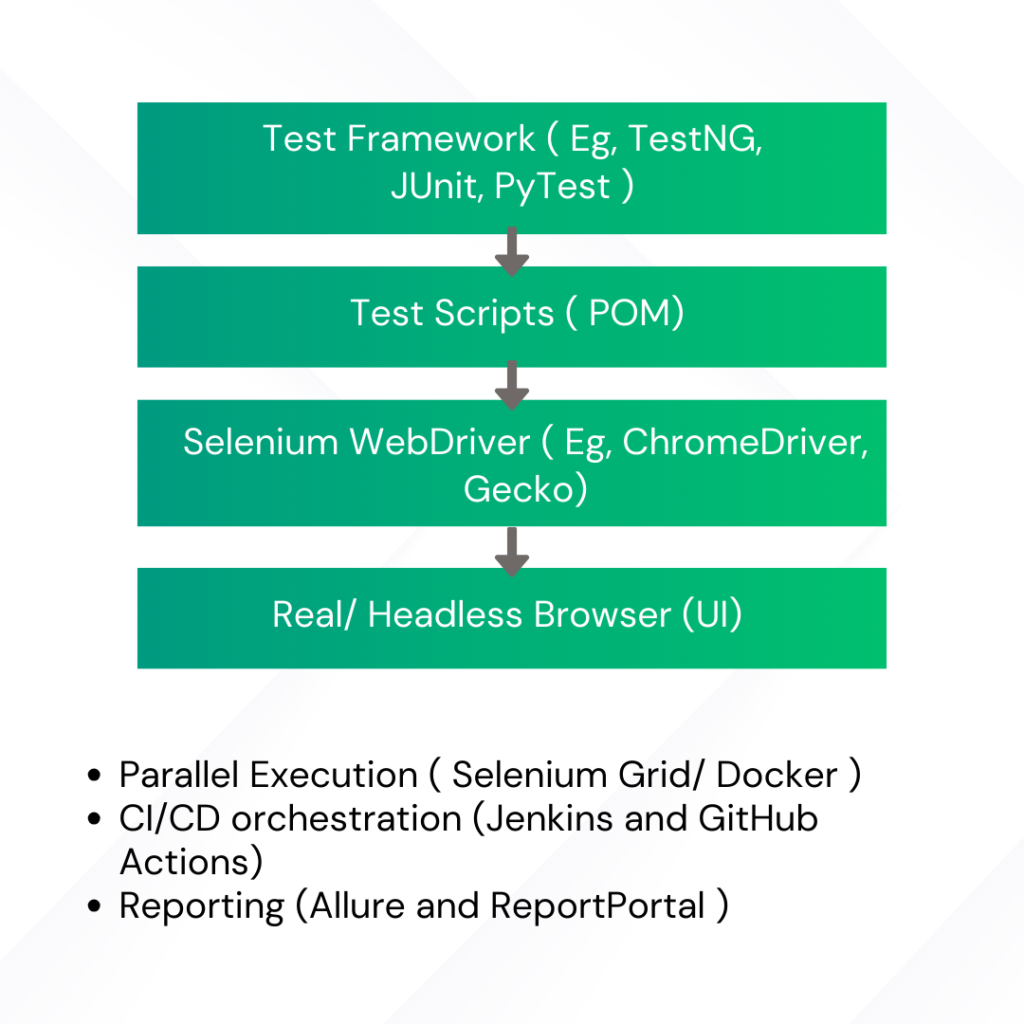
Setting Up Selenium for Scalable UI Testing
For enterprise-grade automation, a basic setup won’t cut it. Here’s a strategic setup plan:
1. Framework Selection
Use TestNG, JUnit (Java), PyTest (Python), or NUnit (C#) as your test framework.
2. Project Architecture
Adopt a modular structure:
- base/
- tests/
- pages/
- utils/
- config/
Use Page Object Model (POM) or Page Factory with service layers to isolate UI interaction from logic.
3. Dependency Management
Use Maven/Gradle for Java or pip/poetry for Python to manage libraries (Selenium, Allure, Faker, etc.).
4. WebDriver Management
With Selenium 4 (Java), driver setup becomes significantly easier thanks to the built-in SeleniumManager, which automatically resolves and manages browser drivers like ChromeDriver and GeckoDriver—removing the need for manual downloads or environment configurations.
For more advanced or legacy scenarios, alternative options are still valuable:
- WebDriverManager (external library): Offers greater control over driver versioning, proxy settings, and compatibility—ideal for CI pipelines, older setups, or when you need custom behavior.
- RemoteWebDriver: Enables test execution on distributed environments like Selenium Grid, or cloud-based platforms such as BrowserStack, Sauce Labs, or containerized setups with Docker.
Best Practice: Use SeleniumManager for modern projects to reduce setup overhead. Opt for WebDriverManager or RemoteWebDriver when you need advanced control, scalability, or compatibility with cloud infrastructure.
5. Environment Configuration
Externalize URLs, browser settings, credentials using config.properties, YAML, or .env files.
Writing a Robust First UI Test
Here’s an advanced example in Java using TestNG and Page Object Model:
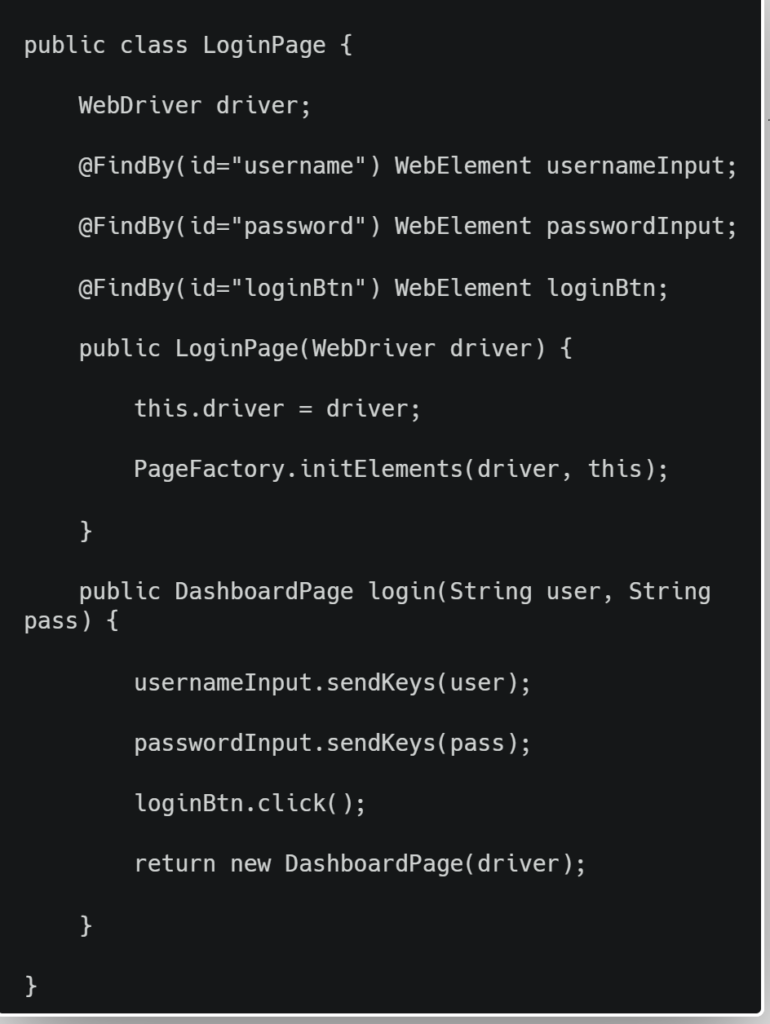
LoginTest.java:
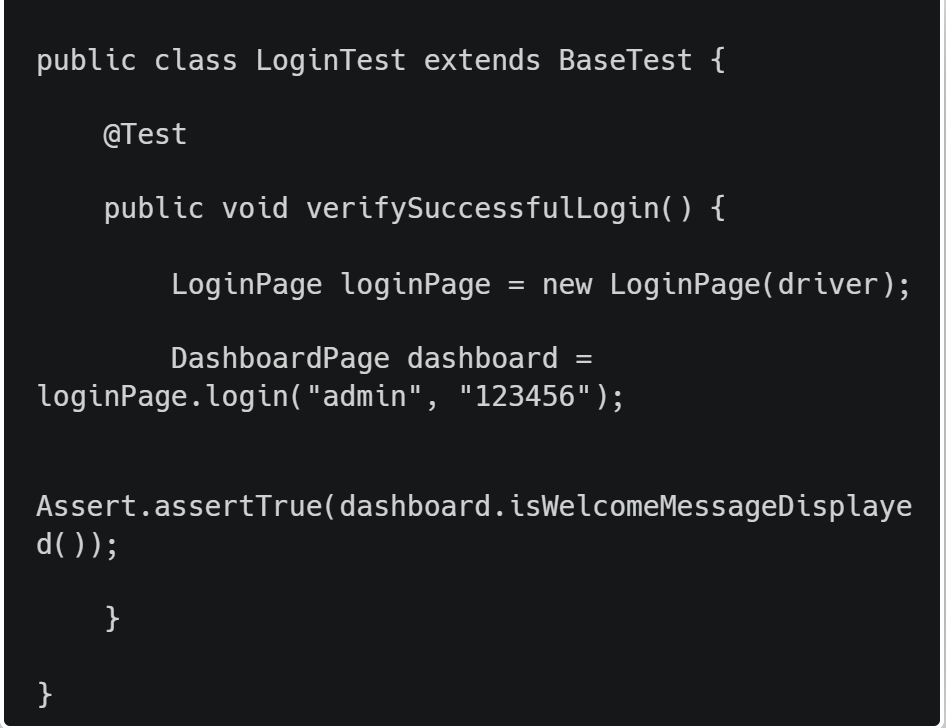
This structure is maintainable, scalable, and integrates easily into pipelines.
Key Challenges in Automated UI Testing with Selenium
Automated UI testing using Selenium, while powerful, faces several real-world hurdles—especially in large-scale, modern web applications. Below are the most pressing challenges QA teams encounter:
1. Dynamic Web Elements and DOM Instability
Web applications built with modern frameworks like React or Angular frequently update DOM elements dynamically. This leads to:
- Elements becoming stale
- Intermittent “element not found” errors
- Test failures due to changing element attributes
2. Test Flakiness and Timing Issues
Flaky tests—ones that fail randomly—are common due to:
- Slow-loading elements
- Backend API delays
- Animations or loaders not accounted for
This reduces confidence in test results and slows down CI/CD pipelines.
3. Fragile and Poor Locator Strategies
Tests that rely on brittle XPath or dynamic IDs (e.g., id-xyz123) often break after UI updates. This leads to:
- High maintenance overhead
- Constant locator updates in test scripts
4. Cross-Browser and Cross-Platform Discrepancies
Selenium supports all major browsers, but different rendering engines (e.g., Blink vs WebKit) can cause:
- Inconsistent test results
- CSS rendering or JavaScript execution differences
5. Scalability and Infrastructure Complexity
Executing tests across multiple browsers and platforms in parallel requires:
- Selenium Grid setup
- Docker or cloud infrastructure
- Complex DevOps configuration
6. Test Data and Environment Dependencies
Tests often fail due to:
- Reliance on real-time production/test data
- Shared or unstable test environments
- Lack of control over data state
7. Limited Native Debugging and Reporting
Selenium lacks out-of-the-box support for:
- Rich reporting
- Screenshots/videos on failure
- Detailed error tracing
Debugging test failures becomes difficult, especially in headless CI environments.
Best Practices: How Selenium Overcomes These Challenges
By applying proven techniques and architectural practices, you can minimize these issues and build a more stable, maintainable UI test suite.
1. Handle Dynamic Elements with Smart Waits and Stable Locators
- Use WebDriverWait and ExpectedConditions to wait for elements dynamically.
- Avoid brittle XPath; prefer CSS selectors or custom attributes like data-testid or aria-label.
- Introduce retry logic or custom wait utilities for complex loading behaviors.
wait.until(ExpectedConditions.visibilityOfElementLocated(By.cssSelector(“[data-testid=’submit-btn’]”)));
2. Stabilize Flaky Tests with Synchronization and Retry Logic
- Avoid Thread.sleep(); use explicit and fluent waits instead.
- Implement test retries only for known flaky cases.
- Use pre/post-condition checks in test scripts (e.g., verify element visibility before interaction).
3. Use Page Object Model (POM) and Robust Locator Strategy
- Implement POM to abstract element locators and user interactions.
- Keep locators maintainable and separate from test logic.
- Review locator strategies periodically to adapt to UI changes without affecting core logic.
4. Execute Cross-Browser Testing Efficiently
- Integrate with Selenium Grid, Docker, or cloud services like BrowserStack or SauceLabs.
- Test critical workflows across multiple browsers early in the CI pipeline.
- Use browser-specific handling where necessary for known compatibility issues.
5. Enable Scalable Infrastructure with Parallel Execution
- Use Docker Compose for running scalable Selenium Grids locally.
- Leverage test orchestration tools like Testcontainers for ephemeral Selenium Grid environments to ensure clean, disposable, and reproducible test setups.
- Configure parallel test execution using TestNG, JUnit, or Pytest.
- Integrate with CI tools like Jenkins, GitHub Actions, or GitLab CI/CD for automated test runs.
Run Selenium UI Tests
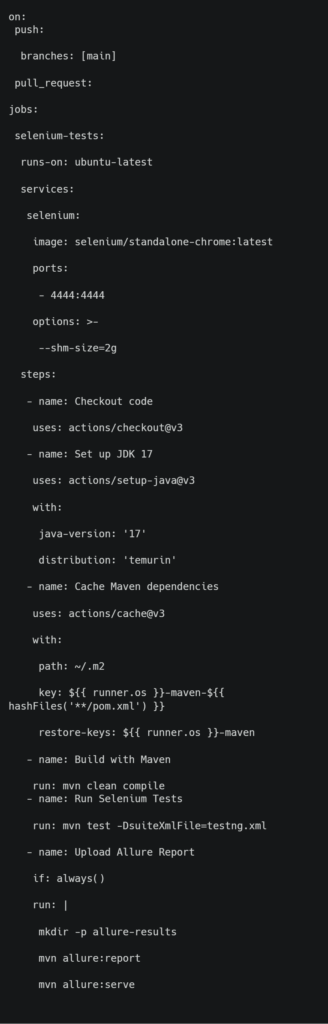
Supports:
- Selenium execution inside a Docker container
- JDK and Maven setup
- Allure reporting
6. Decouple Test Data and Use Mocks Where Possible
- Drive tests using external data sources (CSV, JSON, Excel, or databases).
- Use Faker libraries for generating mock user data.
- Leverage mock servers or service virtualization to simulate backend responses.
7. Integrate Advanced Reporting and Observability Tools
- Integrate Allure, Extent Reports, or ReportPortal for visual test reports, screenshots, and logs.
- Capture screenshots on failure for every test step.
- Store artifacts like logs and screenshots in CI systems for root-cause analysis.
Final Thoughts
Selenium UI testing is not just about automating clicks—it’s about building a test architecture that ensures stability, scalability, and confidence in your releases. By addressing real-world challenges with best-in-class practices, your automation strategy can support modern Agile and DevOps workflows with efficiency.
What’s New in Selenium 4?
Selenium 4 brings several enhancements that modernize and simplify the UI automation process:
- Native support for W3C WebDriver protocol – Ensures better cross-browser compatibility and stability
- Built-in driver management with SeleniumManager – Automates browser driver setup, reducing configuration efforts
- Relative locators for nearby elements – Allows more intuitive element targeting based on spatial relationships
- Enhanced debugging support with CDP integration – Enables access to browser logs, network traffic, and performance metrics
- Improved Selenium Grid with Docker support – Simplifies distributed test execution and scaling in containerized environments
Need help building a scalable, CI-integrated Selenium test framework?
Testrig Technologies has helped startups and enterprises deploy reliable UI automation at speed. Talk to our experts today and take your QA maturity to the next level.